Fasthak
FastHak is a web server written in Go designed for rapid front-end development. It uses Server Sent Events for live-reload, and automatically injects the necessary javascript files, allowing you to get straight to developing your awesome web app. It is a small binary you can drop directly in your project root. You can extend it by leveraging its flexible plug-in architecture.
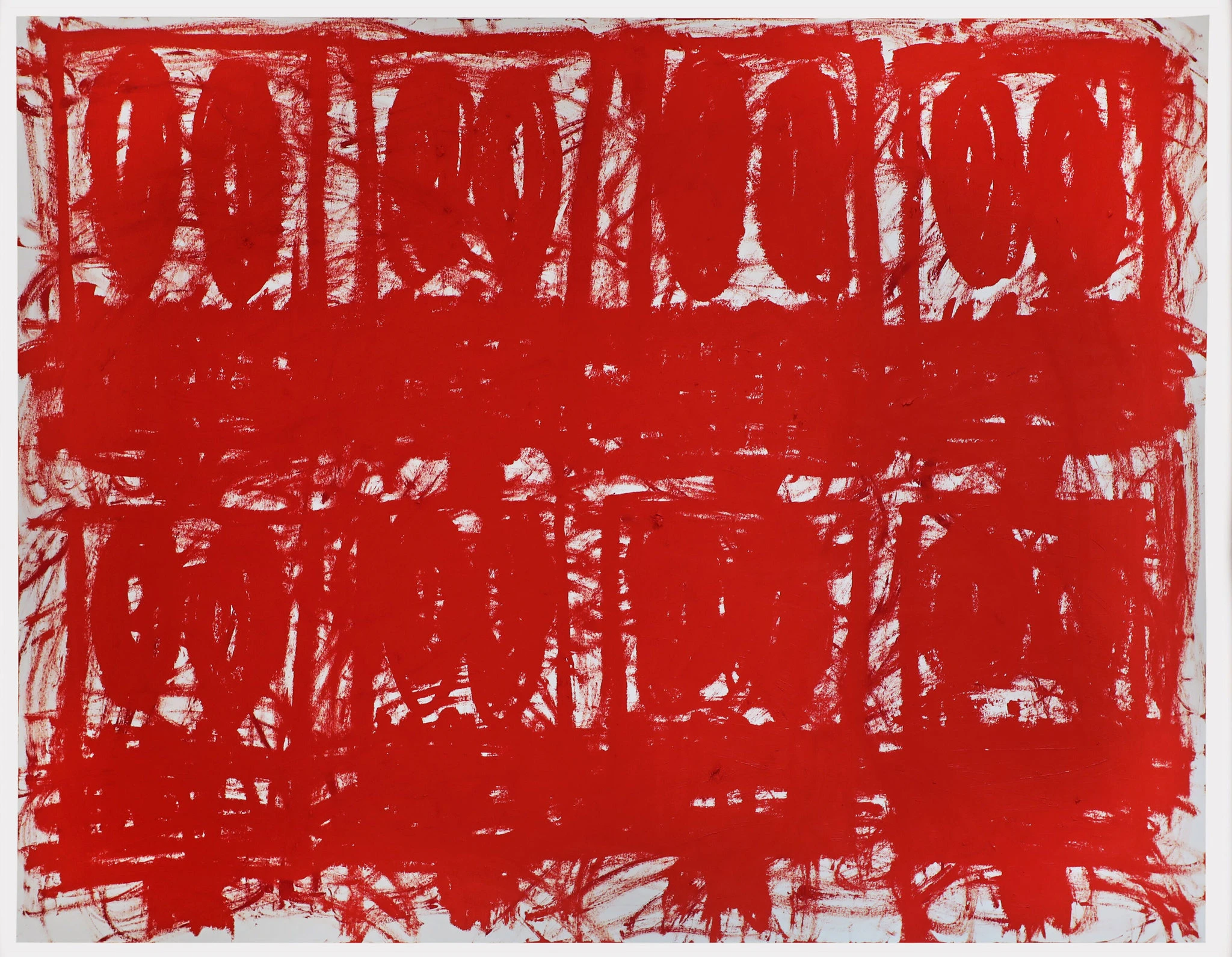
Getting Started
FastHak embeds SSL certificates for rec.la domain, whose subdomain values point to localhost, so your local app will be able to take advantage of a fully secure connection.
Install fasthak:
go install github.com/sean9999/fasthak@latest
assuming you want to start your server against the ./public subdirectory:
fasthak -dir=./public
It will serve your app on https://fasthak.rec.la:9443
, as you might expect. You’ll want to at least have an index.html
there.
The client-side code is available at https://fasthak.rec.la:9443/.hak/js/
. You do not need to include these files in your project. They are embedded in the server itself. You will want to point to them from your project. Example:
index.js
import { hak, registerSSE } from "./.hak/js/sse.js";
Bare Minimum front-end Code
The bare bare minimum to see it in action would be to have one index.html
file that looks like this:
index.html
<!DOCTYPE html>
<html>
<head>
<script defer>
const sse = new EventSource(`/.hak/fs/sse`);
sse.addEventListener('fs', (event) => {
const payload = event.data;
console.log(payload);
});
</script>
</head>
<body>
<p>open console and have a look see</p>
</body>
</html>
But since fasthak provides some convenience functions out the box, you may as well take advantage:
index.js
import { hak, registerSSE } from "./.hak/js/sse.js";
registerSSE().then(sse => {
sse.addEventListener('fs', (event) => {
const payload = event.data;
console.log(payload);
});
});
index.html
<!DOCTYPE html>
<html>
<head>
<script defer src="./index.js"></script>
</head>
<body>
<p>open console and have a look see</p>
</body>
</html>
Of course, that only registers the events. You must choose what to do in response. Here’s what I do. It’s the simplest live-reloader I can think of:
index.js
registerSSE().then(sse => {
sse.addEventListener('fs', (event) => {
// close the channel
// not strictly necessary, but polite
sse.close();
// reload browser window
// sse will reconnect on DOMContentLoaded
window.location.reload();
});
});
Why Server Sent Events?
SSE makes more sense than websockets, which is what traditional live-reloaders use. First off, the information does not need to be two-way. Your app has nothing to say to the server. Your server has much to say to your app. The duplex connection that websockets provide are overkill and wasted resources. Fasthak is more correct and efficient.
Secondly, fasthak provides filesystem events as DOM Custom Events. You choose what you want to do with those events, which in the simplest case is to reload your browser, but could just as easily leverage Hot Module Replacement, or some other action that only you can anticipate. LiveReload has some degree of HMR (stylesheets and images are reloaded via javascript), but it’s brittle on non-configurable. FastHak gives you total control.
How does it work
Rebouncer does all the heavy-lifting. Fasthak simply wraps a static server around it, and emits filesystem events in SSE format.
What’s Next?
Due to it’s design, FastHak could easily be extended to respond to events other than fileSystem events. For example, it could provide introspection capabilities to your otherwise static HTML site, or information about the server such as load and resource usage. There is no reason FastHak could not be used in production.
Additionally, it would be useful to provide hooks for common frameworks, such as React and Vue.
Furthermore, I would like to have a fasthak init
command that automatically generates the minimal scaffolding needed of your static sites.
Finally, the client code should be available as a browser extension, so that it’s injected into the page but not a part of your codebase.
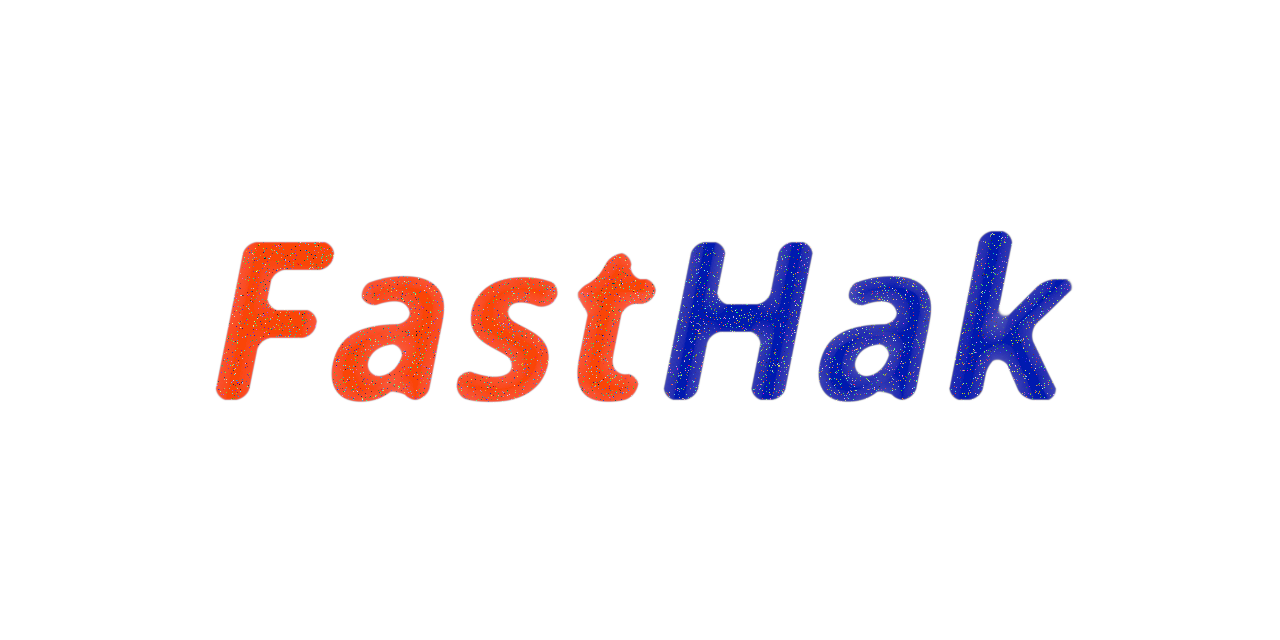